전체 코드
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
public class PlayerController : MonoBehaviour | |
{ | |
// 스피드 조정 변수 | |
[SerializeField] | |
private float walkSpeed; | |
[SerializeField] | |
private float runSpeed; | |
private float applySpeed; | |
[SerializeField] | |
private float crouchSpeed; | |
// 점프 | |
[SerializeField] | |
private float jumpForce; | |
// 상태 변수 | |
private bool isRun = false; | |
private bool isCrouch = false; | |
private bool isGround = true; | |
// 앉았을 때 얼마나 앉을지 결정하는 변수 | |
[SerializeField] | |
private float crouchPosY; | |
private float originPosY; | |
private float applyCrouchPosY; | |
// 땅 착지 여부 | |
private CapsuleCollider capsuleCollider; | |
// 카메라의 민감도 | |
[SerializeField] | |
private float lookSensitivity; | |
// 카메라의 각도의 한계 | |
[SerializeField] | |
private float cameraRotationLimit; | |
private float currentCameraRotationX = 0; | |
// 필요한 컴포넌트 | |
[SerializeField] | |
private Camera theCamera; | |
private Rigidbody myRigid; | |
// Start is called before the first frame update | |
void Start() | |
{ | |
capsuleCollider = GetComponent<CapsuleCollider>(); | |
myRigid = GetComponent<Rigidbody>(); | |
applySpeed = walkSpeed; | |
// 초기화 | |
originPosY = theCamera.transform.localPosition.y; | |
applyCrouchPosY = originPosY; | |
} | |
// Update is called once per frame | |
void Update() | |
{ | |
IsGround(); | |
TryJump(); | |
TryRun(); | |
TryCrouch(); | |
Move(); | |
CameraRotation(); | |
CharacterRotation(); | |
} | |
// 앉기 시도 | |
private void TryCrouch() | |
{ | |
if (Input.GetKeyDown(KeyCode.LeftControl)) | |
{ | |
Crouch(); | |
} | |
} | |
// 앉기 동작 | |
private void Crouch() | |
{ | |
isCrouch = !isCrouch; | |
if (isCrouch) | |
{ | |
applySpeed = crouchSpeed; | |
applyCrouchPosY = crouchPosY; | |
} else | |
{ | |
applySpeed = walkSpeed; | |
applyCrouchPosY = originPosY; | |
} | |
StartCoroutine(CrouchCoroutine()); | |
} | |
// 부드러운 앉기 동작 실행 | |
IEnumerator CrouchCoroutine() | |
{ | |
float _posY = theCamera.transform.localPosition.y; | |
int count = 0; | |
while(_posY != applyCrouchPosY) | |
{ | |
count++; | |
_posY = Mathf.Lerp(_posY, applyCrouchPosY, 0.3f); | |
theCamera.transform.localPosition = new Vector3(0, _posY, 0); | |
if(count > 15) | |
{ | |
break; | |
} | |
yield return null; | |
} | |
theCamera.transform.localPosition = new Vector3(0, applyCrouchPosY, 0f); | |
} | |
// 지면 체크 | |
private void IsGround() | |
{ | |
isGround = Physics.Raycast(transform.position, Vector3.down, capsuleCollider.bounds.extents.y + 0.1f); | |
} | |
// 점프 시도 | |
private void TryJump() | |
{ | |
if (Input.GetKeyDown(KeyCode.Space) && isGround) | |
{ | |
Jump(); | |
} | |
} | |
// 점프 | |
private void Jump() | |
{ | |
// 앉은 상태에서 점프시 앉은 상태 해제 | |
if (isCrouch) | |
{ | |
Crouch(); | |
} | |
myRigid.velocity = transform.up * jumpForce; | |
} | |
// 달리기 시도 | |
private void TryRun() | |
{ | |
if (Input.GetKey(KeyCode.LeftShift)) | |
{ | |
Running(); | |
} | |
if (Input.GetKeyUp(KeyCode.LeftShift)) | |
{ | |
RunningCancel(); | |
} | |
} | |
// 달리기 실행 | |
private void Running() | |
{ | |
if (isCrouch) | |
{ | |
Crouch(); | |
} | |
isRun = true; | |
applySpeed = runSpeed; | |
} | |
// 달리기 취소 | |
private void RunningCancel() | |
{ | |
isRun = false; | |
applySpeed = walkSpeed; | |
} | |
// 움직임 실행 | |
private void Move() | |
{ | |
float _moveDirX = Input.GetAxisRaw("Horizontal"); | |
float _moveDirZ = Input.GetAxisRaw("Vertical"); | |
Vector3 _moveHorizontal = transform.right * _moveDirX; | |
Vector3 _moveVertical = transform.forward * _moveDirZ; | |
Vector3 _velocity = (_moveHorizontal + _moveVertical).normalized * applySpeed; | |
myRigid.MovePosition(transform.position + _velocity * Time.deltaTime); | |
} | |
// 좌우 캐릭터 회전 | |
private void CharacterRotation() | |
{ | |
float _yRotation = Input.GetAxisRaw("Mouse X"); | |
Vector3 _characterRotationY = new Vector3(0f, _yRotation, 0f) * lookSensitivity; | |
myRigid.MoveRotation(myRigid.rotation * Quaternion.Euler(_characterRotationY)); | |
} | |
private void CameraRotation() | |
{ | |
// 상하 카메라 회전 | |
float _xRotation = Input.GetAxisRaw("Mouse Y"); | |
float _cameraRotationX = _xRotation * lookSensitivity; | |
currentCameraRotationX -= _cameraRotationX; | |
currentCameraRotationX = Mathf.Clamp(currentCameraRotationX, -cameraRotationLimit, cameraRotationLimit); | |
theCamera.transform.localEulerAngles = new Vector3(currentCameraRotationX, 0f, 0f); | |
} | |
} |
구현 영상
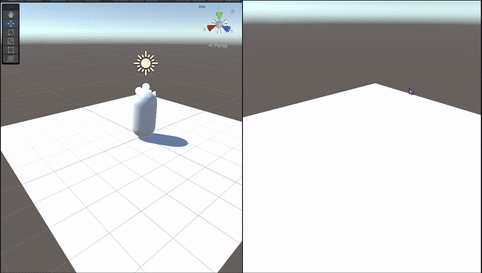
'Unity' 카테고리의 다른 글
Survival_Game01 - 기본 캐릭터 움직이기 (0) | 2022.08.03 |
---|