목차
let vs const
let | const |
가변한 변수 | 불변한 변수 |
화살표 함수
아래와 같은 코드를 화살표 함수로 바꾸면
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const name = "Lim"; | |
let age = 17; | |
const hasHobbies = true; | |
age = 27; | |
const summariseUser = function(userName, userAge, userHasHobbies) { | |
return ( | |
"Name is " + userName + | |
"Age is" + userAge + | |
"User has hobbies: " + userHasHobbies | |
); | |
}; | |
console.log(summarizeUser(name, age, hasHobbies)); |
아래의 코드와 같이 됨
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const name = "Lim"; | |
let age = 17; | |
const hasHobbies = true; | |
age = 27; | |
const summariseUser = (userName, userAge, userHasHobbies) => { | |
return ( | |
"Name is " + userName + | |
"Age is" + userAge + | |
"User has hobbies: " + userHasHobbies | |
); | |
}; | |
console.log(summarizeUser(name, age, hasHobbies)); |
이렇게 짧고 간단하게 만들기 때문에 사용
아래는 화살표 함수의 더 많은 예시이다.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const add = (a, b) => a + b; | |
const addOne = a => a + 1; | |
const addRandom = () => 2 + 3; | |
console.log(add(1,2)); | |
console.log(addOne(1)); | |
console.log(addRandom()); |
- add 함수처럼 return문이 하나만 있는 화살표 함수라면 중괄호와 return을 생략 가능
- addOne 함수처럼 인수가 하나라면 괄호를 생략 가능
- addRandom 함수처럼 인수가 없다면 비어있는 괄호 한 쌍을 명시
개체, 속성 및 메서드 작업
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const person = { | |
name: "Lim", | |
age: 27 | |
}; | |
console.log(person) |
- 중괄호로 객체를 생성
- 중괄호 안에는 키-값 쌍이 들어감
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const person = { | |
name: "Lim", | |
age: 27, | |
greet: () => { | |
console.log("Hi, I am " + this.name); | |
}, | |
greet2: function() { | |
console.log("Hi, I am " + this.name); | |
}, | |
greet3() { | |
console.log("Hi, I am " + this.name); | |
} | |
}; | |
person.greet(); | |
person.greet2(); | |
person.greet3(); |
- 위와 같이 객체 내에서 함수 사용 가능
- 여기서 this는 주위 객체를 참조
- .으로 속성 또는 매서드(객체 내의 변수나 함수)를 액세스
- 아래는 위 코드의 실행 결과
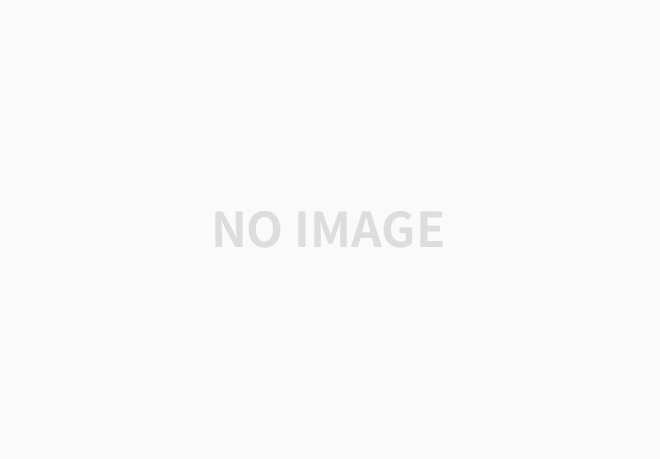
- person.greet()를 실행하면 Hi, I am undefined의 결과를 얻음
- 이는 화살표 함수의 특성때문에 여기서의 this는 이 객체가 아닌 전역 범위, 전역 노드 런타임 범위를 참조함
- 이 객체를 참조하도록 하려면 화살표 함수를 사용하지 않고 기존의 함수를 사용해야 함
배열과 배열 메서드
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const names = ["Lim", "Kim", "Park"]; | |
for (let name of names) { | |
console.log(name); | |
} |
- 위 코드는 for of 루프로 매 반복마다 각 원소를 name 변수로 저장하여 출력
- 아래는 위 코드의 실행 결과

This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const names = ["Lim", "Kim", "Park"]; | |
console.log(names.map(name => { | |
return "Name: " + name; | |
})); |
- 위 코드는 map을 사용하여 새로운 배열을 출력
- 아래는 위 코드의 실행 결과

스프레드 및 레스트 연산자
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const names = ["Lim", "Kim", "Park"]; | |
const copiedNames = names.slice(); | |
const copiedNames2 = [...names]; | |
console.log("copiedNames : " + copiedNames); | |
console.log("copiedNames2 : " + copiedNames2); |
- copiedNames와 같이 slice를 이용하여 배열을 복사
- 인수를 입력해서 복사하길 원하는 원소의 범위를 제한할 수 있음
- copiedNames2와 같이 점 세 개를 이용하여 배열을 복사할 수 있음
- 여기서의 점 세 개(...)는 스프레드 연산자
- 스프레드 연산자는 연산자 뒤에 오는 배열이나 객체를 받아서 원소나 속성을 꺼냄
- 아래는 위 코드의 실행 결과

This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const person = { | |
name: "Lim", | |
age: 27, | |
greet() { | |
console.log("Hi, I am " + this.name); | |
} | |
} | |
const copiedPerson = {...person} |
- 객체도 위의 배열과 마찬가지로 이와 같이 사용
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const toArray = (arg1, arg2, arg3) => { | |
return [arg1, arg2, arg3]; | |
}; | |
console.log(toArray(1, 2, 3)); |
- 이렇게 인수가 적을때에는 arg1, arg2, arg3와 같이 사용할 수 있지만, 인수가 많을때에는 arg1, arg2, arg3, arg4, arg5, ...처럼 모든 인수를 작성해줄 수 없음
- 따라서, 이 코드는 전혀 유연하지 않다는 것을 알 수 있음
- 아래는 위 코드의 실행 결과
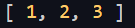
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const toArray = (...args) => { | |
return args; | |
}; | |
console.log(toArray(1, 2, 3, 4, 5)); |
- 여기서의 ...은 레스트 연산자
- 이렇게 레스트 연산자를 사용하면 개수에 관계없이 모든 인수를 가지고 와서 하나의 배열로 나타냄
- 아래는 위 코드의 실행 결과

스프레드 연산자 | 레스트 연산자 |
배열이나 객체에서 원소나 속성을 추출하는데 사용 | 인수 목록이나 함수에서 여러 인수를 하나의 배열로 묶는데 사용 |
구조 분해(Destructuring)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const person = { | |
name: "Lim", | |
age: 27, | |
greet() { | |
console.log("Hi, I am " + this.name); | |
} | |
}; | |
const printName = (personData) => { | |
console.log(personData.name); | |
} | |
printName(person); |
- 위 코드에서 printName이라는 함수는 오직 name만 필요로 함
- 이러한 경우 객체 구조 분해를 사용할 수 있음
- 아래는 위 코드의 실행 결과

This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const person = { | |
name: "Lim", | |
age: 27, | |
greet() { | |
console.log("Hi, I am " + this.name); | |
} | |
}; | |
const printName = ({ name }) => { | |
console.log(name); | |
} | |
printName(person); |
- 구조 분해를 사용하여 name을 추출
- 이 때, 객체의 속성 중 name을 제외한 다른 속성들은 printName 함수에 대해서는 무시
- 이와 마찬가지로 age를 추출할 수도 있고, greet 함수도 추출할 수 있음
- 따라서, 들어오는 객체에서 무엇을 필요로 하는지 어떤 값을 로컬 변수에 저장해서 이 함수에 사용할지 확실히 명시함으로써 이해하기 쉬운 코드를 작성할 수 있음
- 아래는 위 코드의 실행 결과

This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const person = { | |
name: "Lim", | |
age: 27, | |
greet() { | |
console.log("Hi, I am " + this.name); | |
} | |
}; | |
const { name, age } = person; | |
console.log(name, age); |
- 이와 같이 구조 분해를 사용하여 새로운 상수를 생성할 수 있음
- 일반적으로 등호 왼쪽에 중괄호가 있는 경우는 일반적으로 틀린 문법이지만 구조 분해에서는 올바른 방법
- 이 때, 중괄호 안에 입력해야 하는 항목은 person의 속성명과 일치해야 함
- 아래는 위 코드의 실행 결과

This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const names = ["Lim", "Kim", "Park"]; | |
const [name1, name2] = names; | |
console.log(name1, name2); |
- 배열 구조 분해도 객체 구조 분해와 동일
- 배열 구조 분해와 객체 구조 분해의 다른점이라면 배열 구조 분해는 배열 안에 원하는 이름을 선택할 수 있음
- 이는 배열에서는 원소들의 이름이 없고 위치를 기반으로 추출되기 때문
- 따라서, name1은 항상 첫 번째 원소가 되고, name2는 항상 두 번째 원소가 됨
- 아래는 위 코드의 실행 결과

객체 구조 분해 | 배열 구조 분해 |
속성 이름으로 값들을 추출 | 위치를 기반으로 값들을 추출 |
비동기 코드와 프로미스(Promise)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
setTimeout(() => { | |
console.log("Time is done"); | |
}, 1); | |
console.log("Hello"); | |
console.log("Hi"); |
- console.log("Hello")와 console.log("Hi")는 동기화 코드
- console.log("Time is done")은 비동기화 코드
- console.log("Time is done")은 즉시 실행되거나 끝나지 않고 0.001초라는 짧은 시간이라도 시간이 소요되었기 때문
- Node.js와 JavaScript는 일반적으로 코드 실행이 종료될 때까지 멈추지 않기 때문에 여기에서는 콜백 함수라고 하는 함수를 인식하여 모든 동기화 코드를 실행하고 비동기화 코드가 실행됨
- 따라서, 위 코드에서 Time is done이 앞서있더라도 Hello와 Hi를 먼저 보게 됨
- 아래는 위 코드의 실행 결과
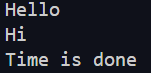
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const fetchData = callback => { | |
setTimeout(() => { | |
callback("Done"); | |
}, 5000); | |
}; | |
setTimeout(() => { | |
console.log("Time is done"); | |
fetchData(text => { | |
console.log(text); | |
}); | |
}, 1000); |
- 위 코드 함수를 콜백하기 위해 텍스트가 전달되어 텍스트를 입력하고 그 텍스트를 console.log를 할 수 있음
- 즉, 두 번째의 fetchData 함수는 콜백으로 전달되고, fetchData에서 이 함수를 실행하게 됨
- 따라서, 위 코드를 실행하게 되면 1초가 지난 뒤에 Time is done이 실행되고, 또 다시 5초가 지난 후에 Done이 실행
- 만약, 중첩된 비동기화 호출이 이보다 더 존재하게 되는 경우, 콜백 시점에서 점점 더 깊게 들어가게 되어 문제에 직면하게 됨
- 그렇기 때문에 프로미스(Promise)라는 기능을 Node.js에서 사용할 수 있음
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const fetchData = () => { | |
const promise = new Promise((resolve, reject) => { | |
setTimeout(() => { | |
resolve("Done"); | |
}, 5000); | |
}); | |
return promise; | |
}; | |
setTimeout(() => { | |
console.log("Time is done"); | |
fetchData().then(text => { | |
console.log(text); | |
return fetchData() | |
}) | |
.then(text2 => { | |
console.log(text2); | |
}); | |
}, 1000); |
- Promise 함수에는 resolve와 reject라는 두 가지 인수를 콜백하게 되는데, resolve는 Promise를 성공적으로 완료, reject는 거부(에러를 표시할 때)할 때 사용
- Promise 함수 안에는 비동기화 코드를 작성
- 대부분의 패키지들은 이미 알아서 진행해 주고 완료된 Promise를 전달하여 배후에서 전부 다 해결해 줌
- 위 코드에서는 setTimeout이 Promise API를 제공하지 않아 콜백 함수를 사용할 수 없어 fetchData()에서는 더 이상 인수를 얻거나 데이터를 가져올 수 없음
- 대신 resolve("Done")을 입력하여 성공적으로 결과값을 반환함
- fetchData()에서 Promise를 정의한 뒤에 반환하기만 하면 되어 return promise를 해줌
- 이 때, return promise는 동기화 코드
- 즉, Promise 내부의 코드가 실행되기 전에 Promise가 생성된 직후 반환 됨
- 이후 fetchData를 호출하는 자리에 더 이상 콜백을 전달하지는 않지만 Promise 상에서 호출할 수 있는 then을 사용하여 Promise를 반환할 수 있음
- then뒤에 단순히 콜백 함수를 정의해서 Promise가 해결된 뒤에 실행되도록 함
- Promise 내부에 블록이 Promise의 일부인 경우 간단히 새로운 Promise를 반환하고 다음 then 블록을 이전 블록 다음에 추가하여 무한히 중첩된 콜백을 두는 것보다 훨씬 읽기 편해짐
- 추가적으로 비동기화 코드를 더 잘 관리할 수 있는 방법 중에는 async, await과 같은 것도 있음
- 아래는 위 코드의 실행 결과

'NodeJS' 카테고리의 다른 글
Node 서버 생성과 라이프사이클 및 이벤트 루프 (0) | 2024.03.26 |
---|---|
웹 작동 방식 (0) | 2024.03.25 |
REPL (0) | 2024.02.22 |
Node.js의 역할 (0) | 2024.02.22 |
Node.js 실행해보기 (0) | 2024.02.22 |