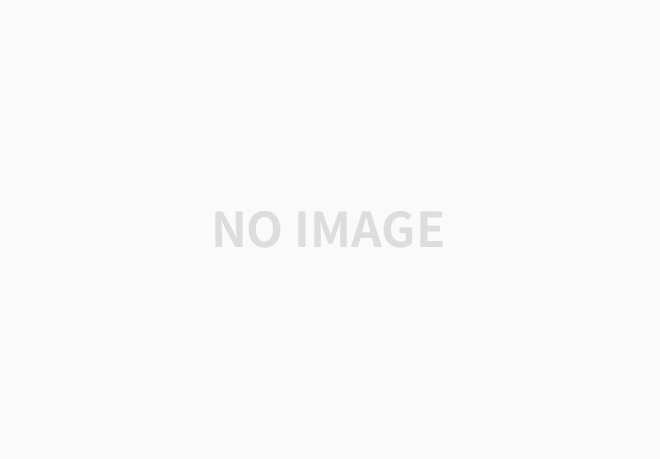
텍스트 보간법
데이터 바인딩
데이터 바인딩의 가장 기본형태는 {{ data }}를 사용하는 것이다.
{{ }} 안에는 data뿐만 아니라 JavaScript 표현식도 사용 가능하다.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<template> | |
<div> | |
<p>문자열 : {{ message }}</p> | |
</div> | |
</template> | |
<script> | |
import { ref } from "vue"; | |
export default { | |
setup() { | |
const message = ref("Hello"); | |
return { | |
message, | |
}; | |
}, | |
}; | |
</script> | |
<style></style> |
{{ message }}와 같이 사용하면 컴포넌트 인스턴스의 message 값으로 대체된다.
message 속성이 변경될 때마다 갱신된다.
v-once
한 번만 렌더링을 하고 데이터가 변경되어도 갱신되지 않게하기 위해 사용한다.
일회성 보간을 수행한다.
예시
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<p v-once>{{ message }}</p> |
두 데이터 바인딩의 차이
예시
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<template> | |
<div> | |
<h1>보간법 차이</h1> | |
<p>{{ message }}</p> | |
<p v-once>{{ message }}</p> | |
<br /> | |
<button v-on:click="message = message + '!'">클릭</button> | |
</div> | |
</template> | |
<script> | |
import { ref } from "vue"; | |
export default { | |
setup() { | |
const message = ref("Hello"); | |
return { | |
message, | |
}; | |
}, | |
}; | |
</script> | |
<style scoped></style> |
⬇️⬇️ 실행 결과 ⬇️⬇️
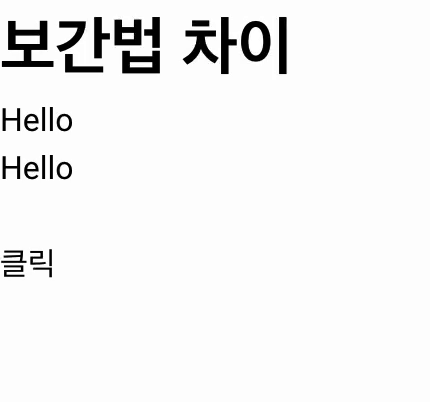
v-html
v-html 디렉티브를 이용하여 실제 HTML로 출력할 수 있다.
웹사이트에서 임의의 HTML을 동적으로 렌더링하면 XSS 취약점으로 이어질 수 있어서 신뢰할 수 있는 콘텐츠에서만 사용하여야 한다.
예시
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<template> | |
<div> | |
<h1>HTML로 출력하기</h1> | |
<p>{{ message }}</p> | |
<p v-html="message"></p> | |
</div> | |
</template> | |
<script> | |
import { ref } from "vue"; | |
export default { | |
setup() { | |
const message = ref("<b>Hello</b>"); | |
return { | |
message, | |
}; | |
}, | |
}; | |
</script> | |
<style scoped></style> |
⬇️⬇️ 실행 결과 ⬇️⬇️
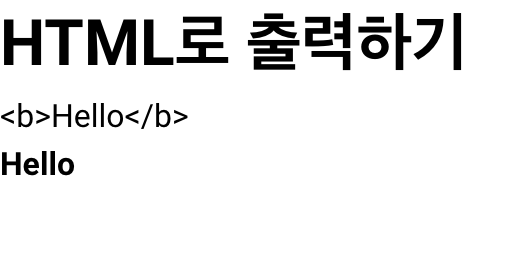
v-bind
{{ }}는 HTML 속성에 사용할 수 없기 때문에 {{ }} 대신 사용한다.
:는 v-bind의 단축 문법이다.
예시
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<template> | |
<div> | |
<h1>v-bind</h1> | |
<div v-bind:title="bindingTitle">제목</div> | |
</div> | |
</template> | |
<script> | |
import { ref } from "vue"; | |
export default { | |
setup() { | |
const bindingTitle = ref("Hi"); | |
return { | |
bindingTitle, | |
}; | |
}, | |
}; | |
</script> | |
<style scoped></style> |
⬇️⬇️ 실행 결과 ⬇️⬇️
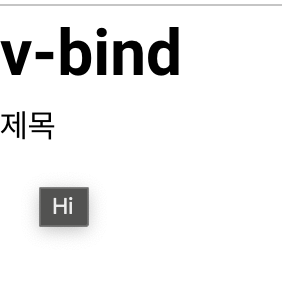
예시: 단축 문법 사용하기
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<template> | |
<div> | |
<h1>v-bind</h1> | |
<div :title="bindingTitle">제목</div> | |
</div> | |
</template> | |
<script> | |
import { ref } from "vue"; | |
export default { | |
setup() { | |
const bindingTitle = ref("Hi"); | |
return { | |
bindingTitle, | |
}; | |
}, | |
}; | |
</script> | |
<style scoped></style> |
예시: 다중 속성 한까번에 바인딩하기
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<template> | |
<div> | |
<h1>v-bind</h1> | |
<input :="attrs" /> | |
</div> | |
</template> | |
<script> | |
import { ref } from "vue"; | |
export default { | |
setup() { | |
const attrs = ref({ | |
type: "password", | |
value: "123456", | |
disabled: false, | |
}); | |
return { | |
attrs, | |
}; | |
}, | |
}; | |
</script> | |
<style scoped></style> |
⬇️⬇️ 실행 결과 ⬇️⬇️
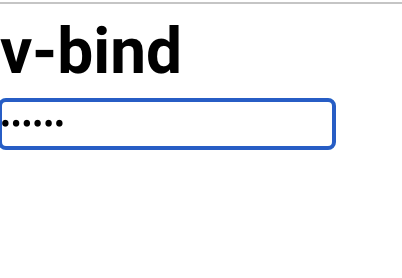
'Vue' 카테고리의 다른 글
Reactivity(반응형) (0) | 2024.07.02 |
---|---|
setup() 함수 (0) | 2024.06.20 |
Composition API (1) | 2024.06.17 |
컴포넌트 (0) | 2024.06.14 |
Vue란 ? (0) | 2024.06.12 |